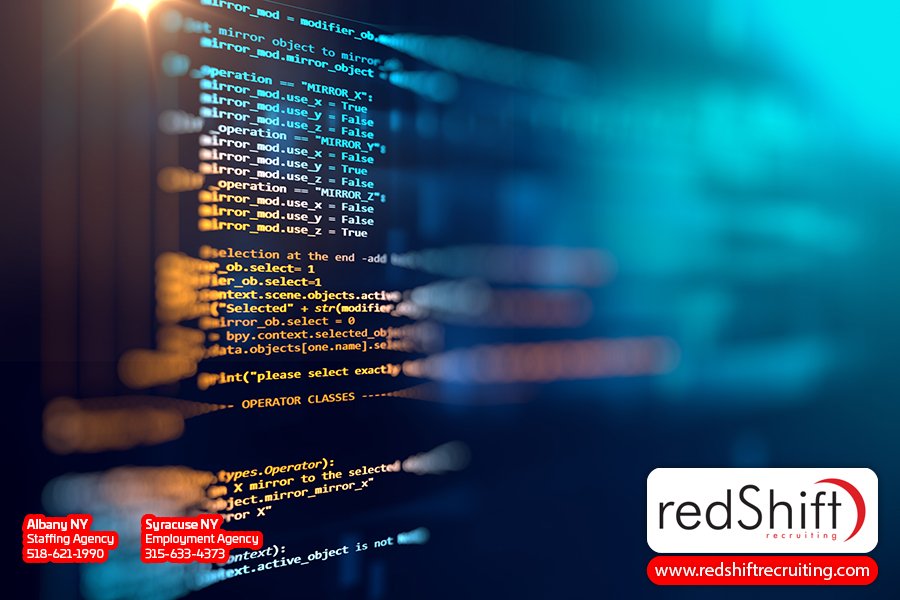
Javascript Functions Every Web Developer Should Know: Getelementbyid() and Addeventlistener()
Are you a web developer looking to enhance your JavaScript skills? If so, then you're in the right place.
In this article, we will dive into two essential functions that every developer should know: getElementById() and addEventListener(). These functions are powerful tools that allow you to manipulate HTML elements and handle events on your web page.
By understanding how to use these functions effectively, you'll be able to create interactive and dynamic websites that engage your users.
So let's get started!
Introduction to JavaScript
In web development, it's essential to understand the basics of JavaScript functions like getElementById() and addEventListener(). These functions play a vital role in manipulating elements on a webpage and adding interactivity.
A JavaScript function is a block of code that performs a specific task. It consists of a function keyword followed by a name, parentheses, and curly braces enclosing the function body. The function body contains the code that will be executed when the function is called.
You can call a function by using its name followed by parentheses. Function parameters are variables that you can pass into a function to customize its behavior. Default parameters allow you to set default values for parameters if no value is provided.
There are different ways to declare functions, including using the arrow function syntax or the traditional function declaration syntax.
Understanding these concepts will help you become proficient in JavaScript programming and enable you to create interactive websites with ease.
Understanding getElementById()
To understand getElementById(), you can access elements in your HTML document by their unique IDs. This function allows you to manipulate and modify the content of these elements using JavaScript.
Here are five key points to help you grasp the concept:
Function declarations: You define a function using the keyword 'function' followed by the function name.
Arrow function syntax: An alternative way to declare functions using a shorter syntax with an arrow (=>) instead of the 'function' keyword.
Function is called: To execute a function, you simply call it by its name, followed by parentheses.
Default values: Functions can have parameters with default values assigned to them, allowing for flexibility in their usage.
Function returns: A function can return a value back to where it was called from, which can be useful for further processing.
Exploring addEventListener()
Try using the addEventListener() function to attach event listeners to elements in your HTML document for enhanced interactivity. This powerful JavaScript function allows you to respond to user actions, such as clicks or key presses, and trigger specific tasks or blocks of code.
As a web developer, understanding and utilizing addEventListener() is crucial for building interactive websites.
With addEventListener(), you can specify the type of event you want to listen for, such as 'click' or 'keydown', and define a function that will be executed when that event occurs. The beauty of this function lies in its ability to handle multiple events on the same element, without overwriting previous ones with the same name.
To use addEventListener(), simply pass in two parameters: the type of event and a callback function that will be executed when the event is triggered. This allows you to create dynamic and engaging web experiences by responding to user interactions effectively.
Working with getElementById() and addEventListener() Together
When combining getElementById() and addEventListener(), you can create dynamic web interactions that enhance user experiences. These two JavaScript functions are essential in web development, allowing you to manipulate HTML elements and respond to user actions.
Here's how they work together:
Use getElementById() to target a specific element on your webpage.
Call addEventListener() on that element to listen for a specific event, such as a click or hover.
Define the function syntax within addEventListener(), either with an anonymous function or by referencing a predefined callback function.
Within this function, you can access global variables or use the return statement to pass values between functions.
Consider adding input parameters to your functions for more flexibility and customization.
Event Handling and Callback Functions
Event handling and callback functions work together to create dynamic web interactions and enhance user experiences. When an event occurs, such as a button click or mouse movement, event handling allows you to respond to that event by executing a specific piece of code.
This is where callback functions come into play. A callback function is a function that is passed as an argument to another function and gets executed when a certain condition is met. Callback functions can be defined using arrow functions, anonymous functions, or even regular named functions. They allow you to customize the behavior of your code based on the specific event that occurred.
For example, you can create a greet function as a callback for a button click event, which will display a greeting message when the button is clicked.
Best Practices for Using getElementById() and addEventListener()
To efficiently select and target elements in your web application, you can use the getElementById() method and addEventListener() function. These JavaScript functions are essential for any web developer looking to enhance their event handling capabilities. Here are some best practices to consider when using these functions:
Choose meaningful and descriptive IDs for your elements.
Use camel case or kebab case for ID names, depending on your preference.
Contextually choose the appropriate event type for addEventListener().
Always pass a callback function as the second argument in addEventListener().
Remember to remove event listeners when they are no longer needed.
By following these best practices, you can ensure that your code remains clean, organized, and efficient.
Incorporating getElementById() and addEventListener() properly will make it easier for you to manipulate elements within your web application and create a seamless user experience.
Common Mistakes and Troubleshooting Tips
One mistake you might encounter is failing to pass a function as the callback argument when using addEventListener(). This can occur when you forget to include the parentheses after the function name.
For example, instead of writing `element.addEventListener('click', greet)`, you may accidentally write `element.addEventListener('click', greet())`. In this case, the greet function will be immediately invoked and its return value will be passed as the callback argument, rather than passing the actual function itself.
To fix this issue, simply remove the parentheses when passing a regular function as a callback. By doing so, you are indicating that you want to pass the actual function reference, not its return value.
Remember that functions in JavaScript can be treated as variables and can be passed around just like any other variable. When using addEventListener(), it is important to provide a valid function as the second argument so that it can be executed when the event occurs.
Case Studies: Real-world Examples of Function Usage
When you're working on a website or web application, it's helpful to study real-world examples of how functions are used to enhance user experience and improve performance. Here are some case studies that showcase the practical usage of JavaScript functions like getElementById() and addEventListener():
Website X effectively uses getElementById() and addEventListener() for form validation, resulting in an improved user experience and accurate data submission.
Web application Y successfully implements dynamic content loading using these functions, leading to enhanced page performance and increased user engagement.
By examining these real-world examples, you can gain insights into how to apply these functions in your own projects. Understanding their usage in different scenarios will help you become a more proficient web developer, capable of creating websites and applications that provide a seamless and enjoyable user experience.
Frequently Asked Questions
How Do I Use the Getelementbyid() Function to Select Multiple Elements?
To select multiple elements using the getElementById() function, you need to keep in mind that this function can only return one element with a specific ID.
However, you can still achieve selecting multiple elements by combining it with other functions or techniques. For example, you could use querySelectorAll() to select all elements with a certain class name or tag name, or loop through an array of IDs and call getElementById() for each one.
Can Addeventlistener() Be Used to Listen for Multiple Events on the Same Element?
Yes, `addEventListener()` can be used to listen for multiple events on the same element. This function allows you to specify multiple event types as arguments, separated by commas. By doing so, you can attach multiple event listeners to a single element, each listening for a different event type.
This is useful when you want your code to respond to various user interactions or actions on the webpage.
What Are Some Alternative Methods to Select Elements Besides Getelementbyid()?
To select elements besides getElementById(), you have a few options.
One alternative is using the getElementsByClassName() method, which selects elements based on their class name.
Another option is querySelector(), which allows you to select elements using CSS selectors.
If you need to select multiple elements, you can use querySelectorAll().
Additionally, if you want to select by tag name, there's getElementsByTagName().
These methods provide flexibility in selecting elements based on different criteria.
Is There a Limit to the Number of Event Listeners That Can Be Added to an Element Using Addeventlistener()?
Yes, there is a limit to the number of event listeners that can be added to an element using addEventListener().
The exact limit may vary depending on the browser and system resources.
Generally speaking, it is recommended to keep the number of event listeners reasonable to avoid performance issues.
Excessive event listeners can slow down your web page and make it less responsive.
Therefore, it's important to optimize the usage of addEventListener() and remove unnecessary event listeners when they are no longer needed.
How Can I Remove an Event Listener That Was Added Using Addeventlistener()?
To remove an event listener that was added using addEventListener(), you can use the removeEventListener() function.
This function allows you to specify the type of event, the callback function, and any additional options or parameters.
By passing in these values correctly, you can effectively remove the event listener from the element.
This is useful when you no longer need the event to be triggered or if you want to update or replace the existing event listener with a new one.
Conclusion
You've gained valuable insights into the practical usage of these functions and how they can enhance user experience and improve performance in real-world scenarios.
By understanding the power of getElementById() and addEventListener(), you have unlocked a whole new level of interactive web development.
These functions allow you to dynamically manipulate the content of your webpage, greet users with personalized messages, and add interactivity through event handling.
With just a few lines of code, you can create engaging forms, responsive menus, and dynamic content updates.
As a web developer, mastering these JavaScript functions is crucial for creating websites that not only look great but also provide a seamless user experience.
So go ahead, explore more examples and integrate these functions into your projects to elevate your web development skills to new heights.